In the Build a React application article of this series, I described how you can create a web application based on React and how you can authenticate users against SAS Viya environment from that application. The previous articles in the series walk through integration of SAS Viya Jobs and CAS tables.
This article is the fourth one in the Develop web applications series:
In this article, we will go through the steps to build a page similar to the one we have seen in the previous articles but this time, the data will come directly from the SAS Compute Server. We will reuse the application structure which was created in this Build a React application article. The remainder of this article will focus on the Compute tab. As for the CAS content, we will display two dropdown boxes to select a library and table then a submit button to retrieve the data to populate a table. The result will be similar to this:
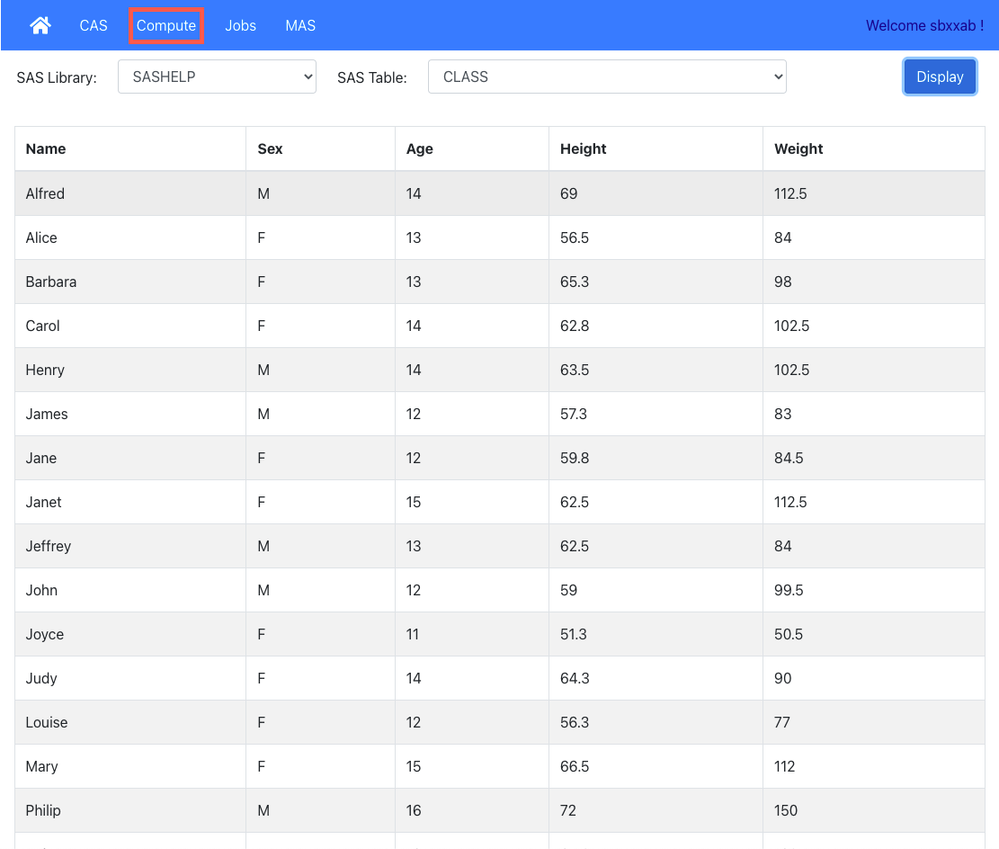
Select any image to see a larger version.
Mobile users: To view the images, select the "Full" version at the bottom of the page.
Reusable components
If you have been through the previous articles in the series, you should know by now that we are building a React application and that one of the major benefits of React is that we can reuse components. In the article covering SAS Viya Jobs and CAS, we have created different components that we will reuse in this article:
- A dropdown box to select a library
- A dropdown box to select a table
- A table that will display the data
- A loader that will be displayed whenever the application is waiting for data from the SAS Viya environment
If you want to see the code for these elements, please check the Reusable components section of the Build a web application using SAS Viya Jobs article.
Specific component for the Compute page
Up till now, the components that we have created so far do not meet the needs for the Compute page. We need to create a specific component: ComputeSelector. This component is a wrapper component that will reuse the LibrarySelector and the TableSelector components. The ComputeSelector is responsible for passing the needed information to the two selectors and to submit the request that will populate the TableViewer which will be added in the next section of this article where we update the Compute page.
Let's create a new file named: ComputeSelector.js. under the components/Selectors folder of our application. The file has multiple sections:
- The import statements This section imports the reusable components we have created and the other components provided natively by React and react-bootstrap. In addition, we are importing the authentication context and the axios instance that we have defined in the previous article of this series.
- The ComputeSelector function definition and the selection handlers This section defines the function and defines the different variables that are needed to get the authentication context, handles the states and defines the functions that are passed to the LibrarySelector and TableSelector components.
- Within the function, the React hook to extract the list of SAS libraries This section handles the request to get the list of SAS libraries. It checks if the user is authenticated and has a Compute Server session. Then it calls the /compute/sessions/${authInfo.session.compute}/data endpoint to extract the list of SAS libraries. When the data is retrieved from SAS Viya, the data is passed to the libraries state.
- Within the function, the React hook to extract the list of tables This section handles the request to get the list of SAS tables. It checks if the user is authenticated and has a Compute Server session. Then it calls the /compute/sessions/${authInfo.session.compute}/data/${selectedLibrary} endpoint to extract the list of SAS tables. When the data is retrieved from SAS Viya, the data is passed to the tables state. Please note that an extra parameter is passed to the endpoint to limit the number of returned rows to 1000. If you are sure that the number of tables will be smaller or higher than this threshold, feel free to adapt the parameter.
- The return statement of the SASSelector function This section defines the layout of the SASSelector component that we will use in next stage. As you can, see the TableSelector will only be enabled when the user has selected a library. The same applies to the Display button which will be only enabled when the user has selected a table.
- The export statement This section is closing the function and exporting the component for later use.

Compute page
Now we have all the components needed to build the Compute page. It is time to update the Compute.js file under pages directory. Once again the file has multiple sections:
- The import statements As earlier, we are here importing all the components that are used in the page.
- The Compute function definition This section defines the function and defines the different variables that are needed to get the authentication context, handles the states and history.
- Within the function, the React hook to validate the authentication The React hook checks whether the user is authenticated or not. If he is not, he is routed to the logon page.
- Within the function, the React hook to handle the Compute Server session In order to access the SAS data, the user needs to be authenticated and to have an active Compute Server session. This section is creating the Compute Server session if there is no session.
- Within the function, the React hook to get the data for the table This hook calls multiple endpoints to:
- Get information about the selected table: /compute/sessions/${authInfo.session.compute}/data/${selection.library}/${selection.table}
- Get the rows for the selected table:/compute/sessions/${authInfo.session.compute}/data/${selection.library}/${selection.table}/rows?limit=${rowCount}
- Get the columns for the selected table: /compute/sessions/${authInfo.session.compute}/data/${selection.library}/${selection.table}/columns?limit=${columnCount}
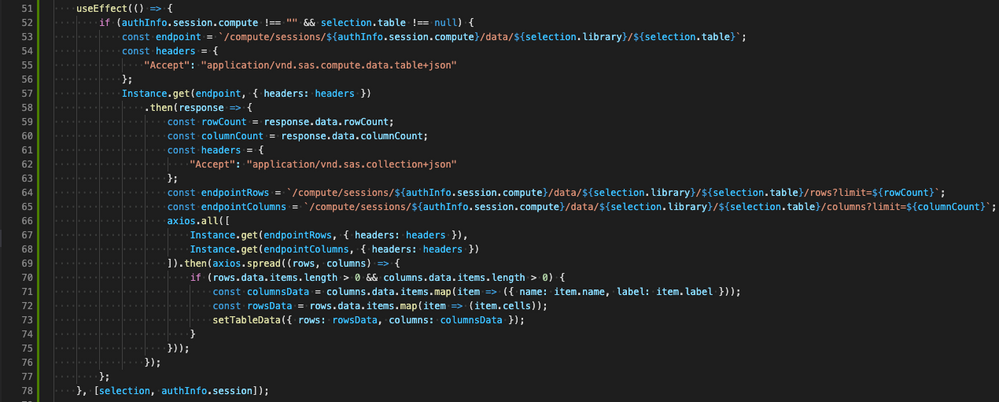
Note: As you can see, we are using the information about the number of rows and number of columns in the URL parameters to ensure that we are retrieving all the columns and all the rows. This can be a bit risky when you don't know the size of the tables. In a real-life scenario, it would be better to limit the number of rows and add some paging functionality to the component. The same applies to the number of rows where it might be a good idea to provide the option for the enduser to select the columns he wants to display.
- The function handling the selection In order to refresh the components, the states for the table data and for the selection are updated. As a side effect, the components are updated and effect hooks are triggered.
- The return statement of the Compute function
- The export statement

Conclusion
In this article, we've seen that by reusing components we have created in the previous articles, we can reduce the number of lines in new pages. If you test the application on your own, you will most probably notice that the time needed to populate the prompts and the result table is smaller compared to the SAS Viya Job but is longer than for the CAS server. The reason is that behind the scenes a Compute Server session is started. So the first request to get the list of libraries might be longer than subsequent requests. If you remember the code, the Compute Server session is reused if it exists. This mechanism allows you to reduce the time for the end-user which can give a better performance impression. As indicated, you should keep in mind that your application might access large tables (number of rows and columns). You can access SAS tables but also RDBMS tables. As a result, you should proactively manage unknown table size in your application to improve the user experience. There are plenty of components available on the web which can help you in that process without the need for you to reinvent the wheel. The code for this article can be downloaded here. This article is the fourth one in the Develop web applications series: